Firebase push notifications are not working in Ionic
Ionic Progressive Web application and integrating the firebase push notification
@ionic/angular application create new project using command
ionic start type=angular
let`s make that straight clear from begin, web Push Notification in Progressive Web App. Regardless of the device lock or unlocked Notifications will only be triggered as long as the application or background.
Configure Firebase in your Project
first add the firebase libraries to your angular or ionic application using the following.
npm install firebase --save
If you not install firebase on your system run this command.
npm install -g firebase-tools
once done, go to you firebase console to configuration of your project w/ firebase. copy the properties.
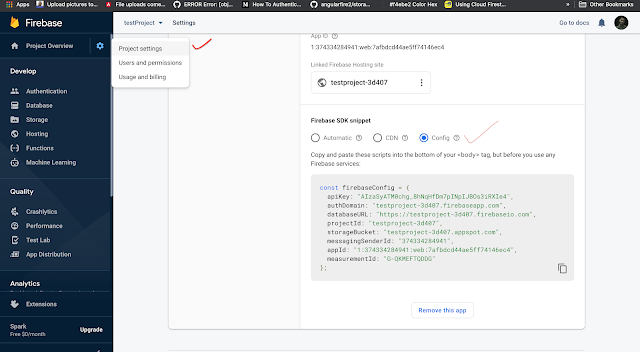
add this configuration to the environment of your project, for example in your environment.ts file.
export const environment = {
const firebaseConfig = {
apiKey: "API_KEY",
authDomain: "testproject firebaseapp.com",
databaseURL: "https://testprojectfirebaseio.com",
projectId: "testproject-3d407",
storageBucket: "testproject-3d407.appspot.com",
messagingSenderId: "msg-sender_id",
}
apiKey: "API_KEY",
authDomain: "testproject firebaseapp.com",
databaseURL: "https://testprojectfirebaseio.com",
projectId: "testproject-3d407",
storageBucket: "testproject-3d407.appspot.com",
messagingSenderId: "msg-sender_id",
}
};
Finally Initialize Firebase in your application's startup, for example in your app.module.ts file.
import { AngularFireModule } from 'angularfire2';
import {environment} from '../environment/environment.ts';
@NgModule({
declarations: [
MyApp,
HomePage
],
imports: [
BrowserModule,
AngularFireModule,
IonicModule.forRoot(MyApp),
// 3. Initialize
AngularFireModule.initializeApp(environment.firebaseConfig),
],
Configure your PWA for FCM
Web push Notifications with FCM, you will need a Web (Project credentials) , server key To generate a new key, open the Cloud Messaging tab of your Firebase console Settings pane and scroll to the Web configuration section.
Finally, to authorize Google FCM to send messages to your application, you will need to add first a fixed value to the Web App Manifest (
manifest.json
or other manifest.webmanifest
) file of your project. {
"gcm_sender_id": "103953800507"
}
"gcm_sender_id": "103953800507"
}
Service Worker
In ionic already create service-worker.js file,
// firebase messaging part: importScripts('https://www.gstatic.com/firebasejs/7.2.1/firebase-app.js'); importScripts('https://www.gstatic.com/firebasejs/7.2.1/firebase-messaging.js'); firebase.initializeApp({ // get this from Firebase console, Cloud messaging section 'messagingSenderId': 'YOURIDFROMYOURFIREBASECONSOLE' }); const messaging = firebase.messaging(); messaging.setBackgroundMessageHandler(function(payload) { console.log('Received background message ', payload); // here you can override some options describing what's in the message; // however, the actual content will come from the Webtask const notificationOptions = { icon: '/assets/images/logo.png' }; return self.registration.showNotification(notificationTitle, notificationOptions); });
Transforming an Ionic Framework app into a PWA is not easy, it is hard work, but we are going to go through every step here. First, go into
src/index.html
and find the below commented out script that enables the service worker and uncomment it:<script>
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('service-worker.js')
.then(() => console.log('service worker installed'))
.catch(err => console.log('Error', err));
}
</script>
Create provider called messaging
run this command
ionic g provider messaging
Request permissions
Obviously you can’t push notifications to a user who would not like to receive such messages. To request his/her agreement you will have then to implement a method which takes care of requesting such permissions.
In messagingProvider.ts
import { Injectable } from "@angular/core"; import { FirebaseApp } from 'angularfire2'; // I am importing simple ionic storage (local one), in prod this should be remote storage of some sort. import { Storage } from '@ionic/storage'; @Injectable() export class messagingProvider {
private messaging; private unsubscribeOnTokenRefresh = () => {}; constructor( private storage: Storage, private app: FirebaseApp ) { this.messaging = app.messaging(); navigator.serviceWorker.register('service-worker.js').then((registration) => { this.messaging.useServiceWorker(registration); //this.disableNotifications() this.enableNotifications(); }); } public enableNotifications() { console.log('Requesting permission...'); return this.messaging.requestPermission().then(() => { console.log('Permission granted'); // token might change - we need to listen for changes to it and update it this.setupOnTokenRefresh(); return this.updateToken(); }); } public disableNotifications() { this.unsubscribeOnTokenRefresh(); this.unsubscribeOnTokenRefresh = () => {}; return this.storage.set('fcmToken','').then(); } private updateToken() { return this.messaging.getToken().then((currentToken) => { if (currentToken) { // we've got the token from Firebase, now let's store it in the database console.log(currentToken) return this.storage.set('fcmToken', currentToken); } else { console.log('No Instance ID token available. Request permission to generate one.'); } }); } private setupOnTokenRefresh(): void { this.unsubscribeOnTokenRefresh = this.messaging.onTokenRefresh(() => { console.log("Token refreshed"); this.storage.set('fcmToken','').then(() => { this.updateToken(); }); }); } }
Comments
Post a Comment